Bootstrapping the project
Tentackle applications are multi module maven projects. However, setting up all poms, directories, plugins, dependencies and rudimentary classes is a complex and errorprone task. Fortunately, Tentackle provides a maven archetype that performs all the necessary steps for you.
To setup the project please follow the instructions here.
In this tutorial we use the following parameters:
- groupId: com.example
- artifactId: tracker
- version: 1.0-SNAPSHOT
- package: com.example.tracker
- application: Tracker
Of course, you can use whatever you like instead.
Let’s see what we’ve got
The created application is meant as a starting point for your project. It does not do very much, except that you can log in and try out some basic things such as creating users, searching and some administrative tasks like changing your password, killing user sessions or configure some security rules. As a first exercise, could you please try to find out how to create a new user and add it to a usergroup via drag’n drop?
Ok, now let’s open the project with the IDE and take a closer look at the project structure. It should look like this:
The following maven modules were generated by the archetype:
- tracker: the project’s super parent
- jlink: parent for the jlink modules (client, daemon, server)
- tracker-client: the FX client
- tracker-common: common classes and resources
- tracker-daemon: the monitoring daemon
- tracker-domain: the implementation of the domain logic
- tracker-gui: the FX user interface
- tracker-pdo: the model definition with the entity interfaces (PDOs)
- tracker-persistence: the implemenation of the persistence logic
- tracker-server: the middle tier server
Why so many modules?
Well, this actually is the bare minimum! The main goal is to separate the different abstraction layers, such as the domain- from the persistence-implementation. The persistence layer is generated from the model and there’s usually not much to code manually, if anything at all. The domain-model and -logic, however, is the heart of the application. This is what makes up your know how! Or to say it in DDD lingo: that’s where we’re coding as close as possible to the so-called ubiquitous language.
You may take a look at the dependency sections of the poms and discover the following relations between the modules:
- tracker-pdo has no dependencies to tracker-domain and tracker-persistence.
- tracker-domain depends on tracker-pdo, but has no dependency to tracker-persistence.
- tracker-persistence depends on tracker-pdo, but has no dependency to tracker-domain.
- tracker-gui depends on tracker-pdo, but has no dependencies to tracker-domain or tracker-persistence (except for test-scope, covered later).
This is also reflected in the module-info files. For example, tracker-pdo:
/** * Persistent Domain Object (PDO) module. */ module com.example.tracker.pdo { exports com.example.tracker.pdo; exports com.example.tracker.pdo.md; exports com.example.tracker.pdo.md.domain; exports com.example.tracker.pdo.md.persist; exports com.example.tracker.pdo.security; exports com.example.tracker.pdo.td; exports com.example.tracker.pdo.td.domain; exports com.example.tracker.pdo.td.persist; requires transitive com.example.tracker.common; provides org.tentackle.common.ModuleHook with com.example.tracker.pdo.service.Hook; }
Ultimately, this means that we’re programming against interfaces rather than concrete classes. The implementations are plugged in as dependencies only when needed at runtime. This happens in tracker-client, tracker-daemon and tracker-server.
As you will learn in a later chapter, the whole framework is built that way, which makes it very easy to extend or replace implementations. It also simplifies writing unit tests a lot.
For an overview of the Tentackle modules, please visit the wiki.
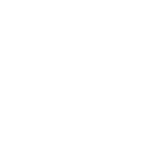
In this tutorial we use IntelliJ IDEA. In principle, you can use any up-to-date Java-IDE or even no IDE at all.
The screenshots for console commands were taken from the Linux bash. MacOS looks pretty similar. For Windows the pathnames must be translated accordingly.
At the time of writing there are the following known quirks or problems with the other IDEs:
- Netbeans 11.x: Navigate to implementation does not work for modular (JPMS) projects
- Eclipse: custom code foldings are not supported